How to Convert a Python List to a Comma-Separated String: Methods and Examples
“Convert a Python list to a comma-separated string with join() for 'a,b,c', map() for integers, or online tools like Online Python List to a Comma-Separated String Converter—perfect for CSV formatting or API data from lists like ['x','y'].”
Why Convert a Python List to a Comma-Separated String?
Turning a Python list like `['apple', 'banana']` into a string like `'apple,banana'` is a common task in programming. Whether you’re preparing data for a CSV file, crafting readable outputs, or feeding an API, knowing how to convert a Python list to a comma-separated string is essential. This guide covers reliable methods—simple enough for beginners, robust enough for pros.
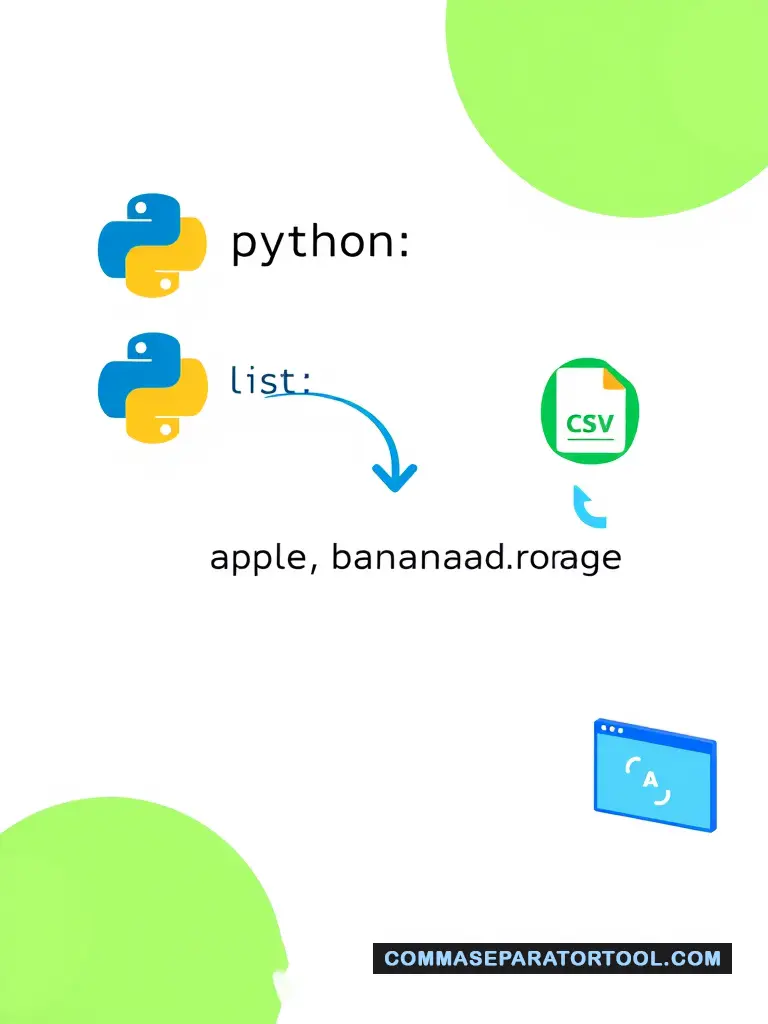
Method 1: Using Python’s join() Method
The Python join method is the go-to for list to string Python conversions.
Steps:
- Start with a list: `fruits = ['apple', 'banana', 'orange']`.
- Use `join()`: `result = ', '.join(fruits)`.
- Print it: `'apple, banana, orange'`.
Code Example:
fruits = ['apple', 'banana', 'orange']
result = ', '.join(fruits)
print(result) # Output: apple, banana, orange
Why it works: `join()` takes an iterable (like a list) and combines its elements into a string, using the separator before `.join()` (here, `', '`). It’s fast and clean—perfect for basic Python list to comma-separated string needs.
Method 2: Handling Non-String Data Types (Integers)
What if your list has integers, like `[1, 2, 3]`? `join()` expects strings, so you’ll need a tweak.
Steps:
- Use a list: `numbers = [1, 2, 3]`.
- Convert to strings with `map()`: `result = ','.join(map(str, numbers))`.
- Result: `'1,2,3'`.
Code Example:
numbers = [1, 2, 3]
result = ','.join(map(str, numbers))
print(result) # Output: 1,2,3
Why it works: `map(str, numbers)` converts each integer to a string, letting `join()` do its job. This is key for mixed data types too.
Method 3: List Comprehension for Customization
Python list comprehension offers flexibility for special cases.
Steps:
- List: `mixed = [1, 'apple', 3.14]`.
- Comprehension: `result = ','.join([str(x) for x in mixed])`.
- Result: `'1,apple,3.14'`.
Code Example:
mixed = [1, 'apple', 3.14]
result = ','.join([str(x) for x in mixed])
print(result) # Output: 1,apple,3.14
Why it works: List comprehension ensures every item is a string, giving you control to filter or format (e.g., rounding floats) before joining.
Method 4: Online Converter Tool
No coding? Use an online Python list to comma separated string converter.
Steps:
- Copy your list: `['x', 'y', 'z']`.
- Paste it into the tool at Comma Separator Tool.
- Get the output: `'x,y,z'`.
Why it works: It’s a quick, free solution for non-programmers or one-off tasks—try it at Comma Separator Tool.
Special Cases
Empty Lists:
empty = []
result = ','.join(empty)
print(result) # Output: '' (empty string)
Nested Lists:
nested = [['a', 'b'], ['c']]
flat = ','.join([item for sublist in nested for item in sublist])
print(flat) # Output: a,b,c
Why it matters: Handling edge cases ensures robust code.
Best Practices
- Efficiency: Use `join()` over loops—it’s faster (O(n) time).
- Readability: Add spaces after commas (`', '`) for human-friendly outputs.
- Type Safety: Always convert non-strings to avoid TypeError.
Real-World Applications
CSV Formatting: Python list to csv string—`['name', 'age']` becomes `'name,age'` for file headers.
API Outputs: Python list to api string—`'id1,id2'` for query parameters.
Readable Displays: `'item1, item2'` for user-facing text.
Conclusion
Converting a Python list to a comma-separated string is straightforward with `join()`, list comprehensions, or tools like Comma Separator Tool. Whether you’re a beginner or pro, these methods—backed by Python Docs—fit any task.
Frequently Asked Questions
Can I use a different separator instead of a comma?
Yes, swap the comma in join()—e.g., `'; '.join(list_name)` for "a; b; c"—to use semicolons or any character.
What’s the fastest method for large lists?
`join()` is the fastest, with O(n) complexity—avoid loops or repeated string concatenation for better performance.
How do I reverse a comma-separated string back to a list?
Use `split()`—e.g., `'a,b,c'.split(',')` returns `['a', 'b', 'c']`—to reverse the process cleanly.
Does join() work with Unicode strings?
Yes, `join()` handles Unicode seamlessly—e.g., `','.join(['α', 'β'])` outputs `'α,β'` without issues.