How to Convert a Comma-Separated String to a List Online
“Convert a comma-separated string to a list online with Python’s split(), JavaScript’s split(), or paste into a Online Comma Separated String to a List Converter, ‘a,b,c’ to [‘a’,‘b’,‘c’] for CSV or app data processing.”
Why Convert a Comma-Separated String to a List Online?
Ever needed to break down a string like "apple,banana,orange" into a list—say, `["apple", "banana", "orange"]`—without digging into heavy coding? That’s where how to convert a comma-separated string to a list online comes in handy. Whether you’re managing input data for applications, processing CSV snippets, or just organizing text, turning a comma-separated string to list online makes it actionable. This guide walks you through practical methods—some code-based, some tool-based—accessible to beginners and pros alike.
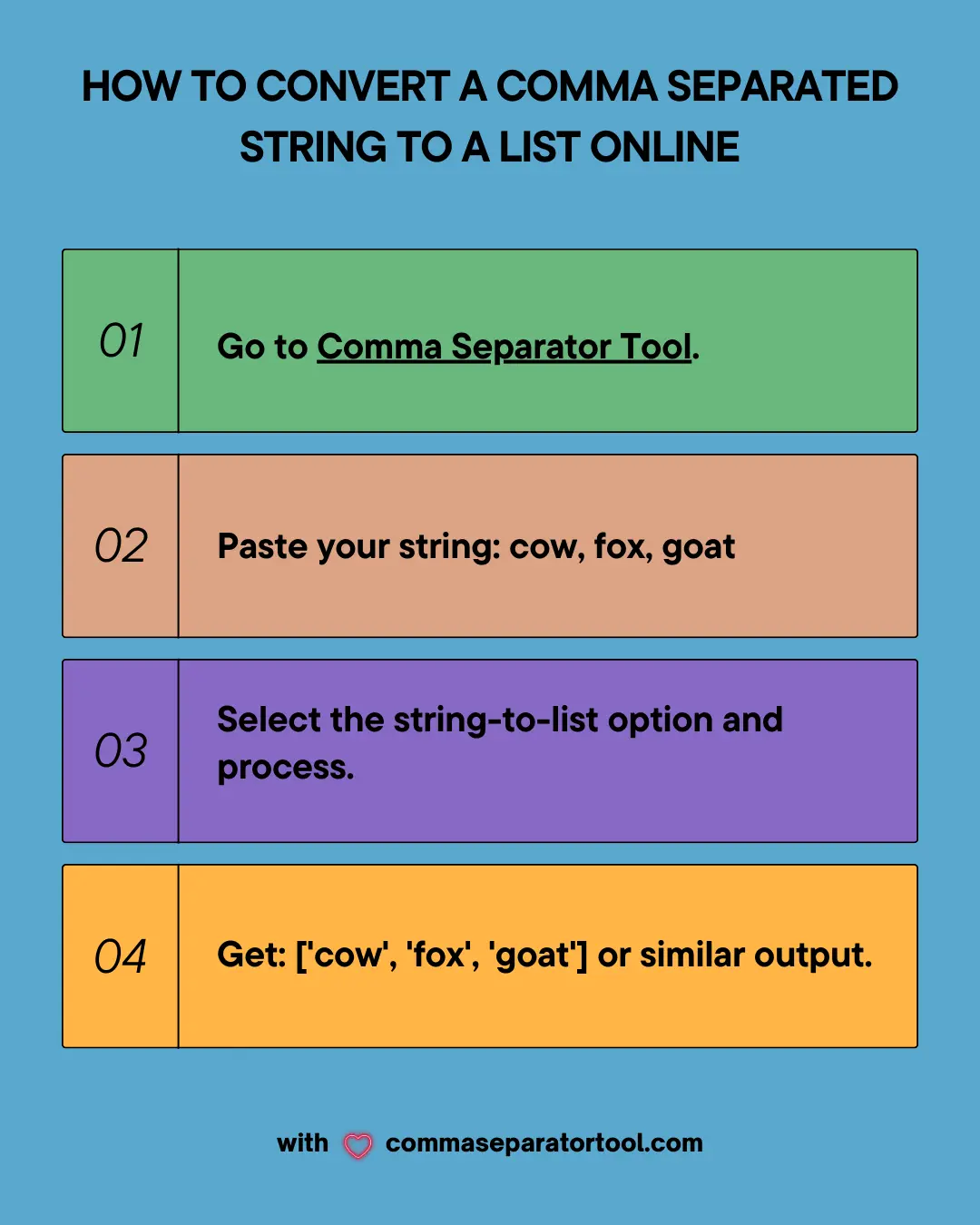
Method 1: Online Tools like Comma Separator
No coding skills? Online common tools like Python comma separated string to list features of Comma Separator Tool make it a breeze.
Steps:
- Go to Comma Separator Tool.
- Paste your string: cow, fox, goat.
- Select the string-to-list option and process.
- Get: ['cow', 'fox', 'goat'] or similar output.
Why it works: This online string to list converter skips the coding step, delivering a list format instantly—ideal for one-off comma-separated string to list online needs.
Method 2: Python’s split() Function
The Python split function is a classic for python comma separated string to list conversions—simple and powerful.
Steps:
- Start with a string: `text = "cat,dog,bird"`.
- Apply `split()`: `my_list = text.split(",")`.
- Result: `["cat", "dog", "bird"]`.
Code Example:
text = "cat,dog,bird"
my_list = text.split(",")
print(my_list) # Output: ['cat', 'dog', 'bird']
Why it works: `split(",")` chops the string at every comma, creating a list instantly—perfect for csv string to list tasks. Newbies love its simplicity; developers rely on its speed.
Method 3: JavaScript’s split() Method
For web developers, the JavaScript split method handles string to list online conversions seamlessly.
Steps:
- Define your string: `let text = "red,blue,green";`.
- Use `split()`: `let myList = text.split(",");`.
- Output: `["red", "blue", "green"]`.
Code Example:
let text = "red,blue,green";
let myList = text.split(",");
console.log(myList); // Output: ['red', 'blue', 'green']
Why it works: It mirrors Python’s approach, splitting at commas for quick comma-separated string to list online results—great for browser-based apps.
Real-World Examples
CSV Data Processing: Convert “name,age,city” into `["name", "age", "city"]` for parsing in Python or JavaScript—think csv string to list for data analysis.
App Inputs: Turn “yes,no,maybe” into `["yes", "no", "maybe"]` for dropdown menus or form handling in apps.
Handling Challenges
Extra Spaces: “cat, dog, bird” becomes `["cat", " dog", " bird"]`—trim with Python’s `text.split(",")` and `strip()`: `my_list = [x.strip() for x in text.split(",")]`. JavaScript: `text.split(",").map(item => item.trim())`.
Special Characters: “cat,dog!,bird” keeps “!”—clean it with regex in Python: `import re; my_list = re.split(",", text.strip("!"))`.
Multiple Delimiters: “cat,dog;bird” needs flexibility—Python: `re.split("[,;]", text)`; JavaScript: `text.split(/[,;]/)`.
Why It Matters
Converting a comma-separated string to list online isn’t just a trick—it’s a bridge to data processing list workflows. Lists are easier to manipulate than strings, whether you’re filtering CSV data or feeding an API. Online tools like Comma Separator make it accessible; coding gives you control.
Frequently Asked Questions
Can I convert a string with no commas into a list?
Yes, split on spaces—e.g., Python: `'cat dog'.split()` gives `['cat', 'dog']`; JS: `'cat dog'.split(' ')` does the same.
What’s the fastest method for large strings?
Python’s `split()` or JS’s `split()` are O(n) and fastest—online tools may lag with huge data due to upload time.
How do I handle empty strings in the conversion?
Python: `''.split(',')` returns `['']`; JS: `''.split(',')` returns `['']`. Filter out empties with list comprehension or `.filter()`.
Are online tools secure for sensitive data?
Check tool privacy policies—most don’t store data, but for sensitive strings, stick to local Python/JS coding.
Conclusion
From Python’s split function to JavaScript’s split method to the online comma separator tool at commaseparatortool.com, converting a comma-separated string to a list online is within reach for all. Pick your method—code it or click it—and turn strings into workable lists effortlessly.